Sbus converter using Arduino
R1 : 10 kOhm,R2 : 4.8 kOhm, Transistor 2N3904 or similar
Therefore you will need an inverter like the one above in order for the Arduino to read it correct.
Arduino sketch
This sketch wil read the Sbus signal and print the first 4 channels, and control the servo on channel 6, that are connectedto pin 14 and Arduino.
The Sbus is connected to the receiver through the inverter shown above.
You will need an USB/Serial converter connected to pin 11 to see the result. This is because the Arduino only have one serial port.
#include <FUTABA_SBUS.h> #include <Servo.h> #include <SoftwareSerial.h> SoftwareSerial mserial(10, 11); // RX, TX FUTABA_SBUS sBus; Servo myservo; void setup(){ mserial.begin(115200); sBus.begin(); myservo.attach(14); } void loop(){ //mserial.println("Loop"); delay(100); sBus.FeedLine(); if (sBus.toChannels == 1){ sBus.UpdateServos(); sBus.UpdateChannels(); sBus.toChannels = 0; mserial.print(sBus.channels[1]); mserial.print("\t"); mserial.print(sBus.channels[2]); mserial.print("\t"); mserial.print(sBus.channels[3]); mserial.print("\t"); mserial.println(sBus.channels[4]); myservo.writeMicroseconds(sBus.channels[6]+800); } }
Note: The serial print and delay should be removed when you are finished debugging. It will cause the servo to jitter
Things to consider
FrSky are making a S-Bus to PWM decoder.
You can connect 4 servo's and you decide which channels should be used by using a FrSky channel-changer.
The framerate of the decoded channels are 9 ms, which can be considered a good thing.
But not all standard analog servos are able to use this high framerate, and therefore some clever devices are needed
See RCMF for an example.
It seems to be much simpler to use the ARduino decoder as it use the good old framerate 20 ms.
This picture shows the framerate from the FrSky converter
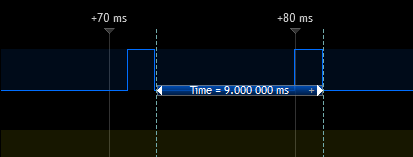
Links
S-Bus decoder by mstrens
S-Bus library for Arduino
S-Bus library presented on Arduino forum
OpenTX on Arduino Mega
Sbus generator
S-Port simulator
S-Port telemetry library
Mike's sbus sketch
Mike's analog Sport for X8R